Hibernate Tips: What’s the Difference Between @Column(length=50) and @Size(max=50)
Take your skills to the next level!
The Persistence Hub is the place to be for every Java developer. It gives you access to all my premium video courses, monthly Java Persistence News, monthly coding problems, and regular expert sessions.
Hibernate Tips is a series of posts in which I describe a quick and easy solution for common Hibernate questions. If you have a question for a future Hibernate Tip, please post a comment below.
Question:
Some of our entity attributes are annotated with @Column(length=50), others with @Size(max=50) and others with both of these annotations.
What’s the difference between the two annotations? Does it make any difference which one we use?
Or do we need both of them?
Solution:
Even though it might seem like both annotations do the same, there are a few crucial differences:
- The @Colum annotation is part of the JPA specification, while the @Size annotation belongs to the BeanValidation specification. So, if you want to use the @Size annotation, you need to add an additional dependency to your project.
- The length attribute of the @Column annotation specifies the size of the database column and gets only used when your persistence provider generates the database schema. But the @Size annotation tells your BeanValidation implementation to perform a proper validation at runtime.
- If you use the @Column annotation and let your persistence provider generate the database schema, your database will reject any values that are more than 50 characters long. The @Size annotation gets evaluated within your Java application before the entity gets persisted or updated.
Let’s take a look at an example.
Using the @Column(size=50) Annotation
If you annotate the title attribute of a Book entity with @Column(size=50), the only thing Hibernate will do it to generate a CREATE TABLE statement that limits the size of the column to 50 characters.
@Entity public class Book { @Id @GeneratedValue private Long id; @Column(length=50) private String title; ... }
create table Book ( id int8 not null, title varchar(50), version int4 not null, primary key (id) )
If you use that CREATE TABLE statement to create your database table, the database will return an error if you try to store more than 50 characters in a title field. But Hibernate will not perform any validation within your application.
Using the @Size(max=50) Annotation
Let’s replace use the @Size(max=50) annotation instead of the previously used @Column annotation.
@Entity public class Book { @Id @GeneratedValue private Long id; @Size(max=50) private String title; ... }
As I explained in great details in a previous article, the JPA and the BeanValidation specification integrate very well. In this case, that provides 2 main benefits:
- If you configure Hibernate to generate the database tables, it will limit the size of the database column based on the maximum size defined by the @Size annotation. As a result, you get the same CREATE TABLE statement as in the previous example.
- Hibernate will automatically trigger your BeanValidation implementation before it persists or updates an entity. That gives you another layer of protection that doesn’t rely on the execution of a specific, generated DDL statement.
Which One Should You Use? @Column(size=50) or @Size(max=50)?
As you’ve seen in the previous paragraphs, Hibernate generates the same CREATE TABLE statements for both annotations. But for the @Size annotation, Hibernate automatically triggers a validation before it inserts or updates the entity.
That makes the @Size annotation the safer and more powerful approach to validate the size of the value of an entity attribute.
Learn more:
To learn more about BeanValidation and its integration with JPA, please take a look at the following articles:
- How to automatically validate entities with Hibernate Validator
- Hibernate Tips: Validate that only 1 of 2 associations is not null
- Hibernate Tips: How to validate that an entity attribute value is within a defined range
- Hibernate Tips: What’s the difference between @Column(nullable = false) and @NotNull
Hibernate Tips Book
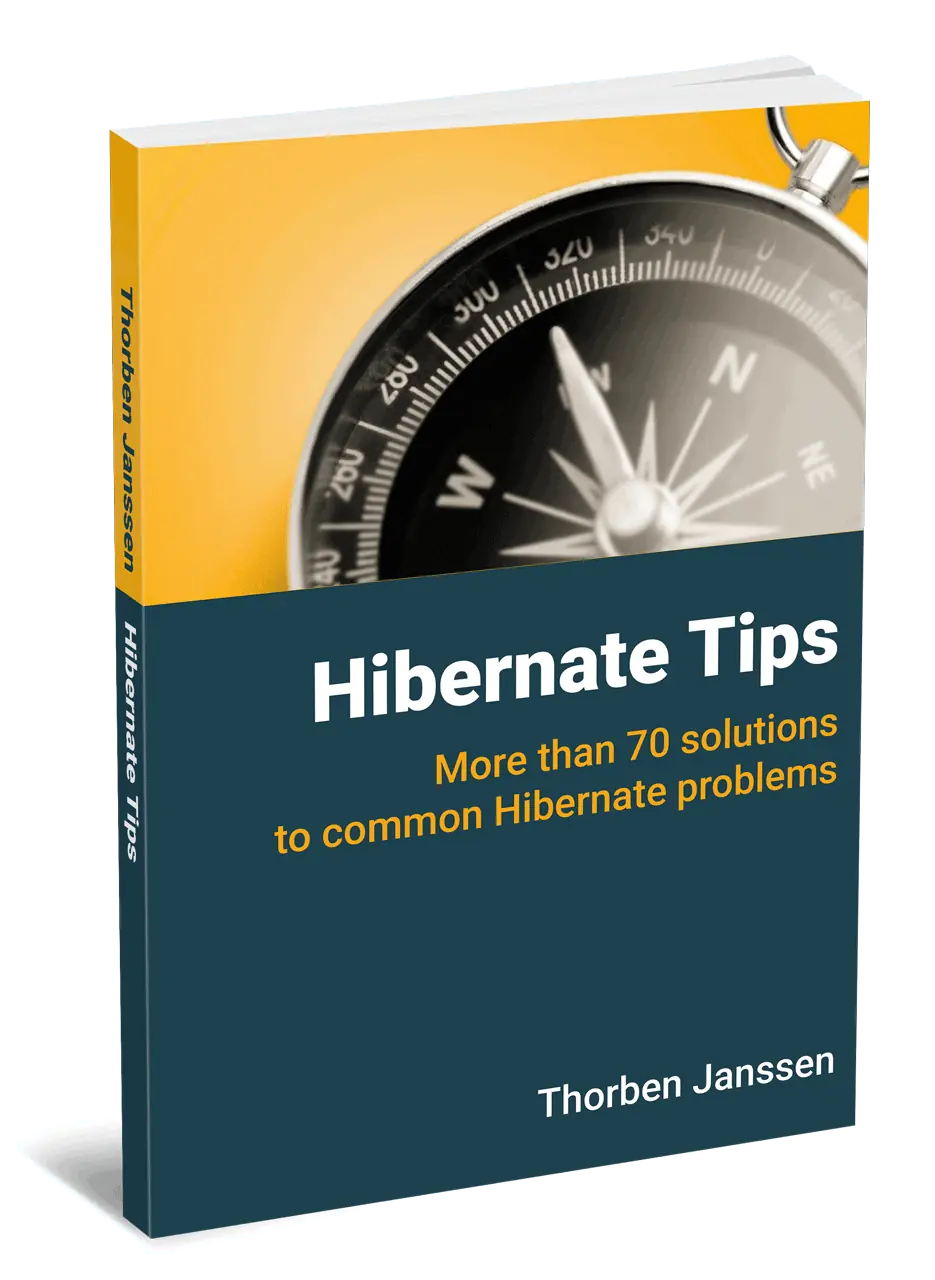
Get more recipes like this one in my new book Hibernate Tips: More than 70 solutions to common Hibernate problems.
It gives you more than 70 ready-to-use recipes for topics like basic and advanced mappings, logging, Java 8 support, caching, and statically and dynamically defined queries.
Thanks for giving useful content.any java related content.we need