Hibernate Tips: Use query comments to identify a query
Take your skills to the next level!
The Persistence Hub is the place to be for every Java developer. It gives you access to all my premium video courses, monthly Java Persistence News, monthly coding problems, and regular expert sessions.
Hibernate Tips is a series of posts in which I describe a quick and easy solution for common Hibernate questions. If you have a question you like me to answer, please leave a comment below.
Question:
My application performs a lot of similar queries and I need to find the output of a specific query in my log file. Is there any way to identify a specific query in the log output.
Solution:
Hibernate can add a comment when it generates an SQL statement for a JPQL or Criteria query or executes a native SQL query. You can see it in your application log file, when you activate SQL statement logging and in your database logs.
You need to activate SQL comments by setting the configuration parameter hibernate.use_sql_comments to true. The following code snippet shows an example configuration in the persistence.xml file.
<?xml version="1.0" encoding="UTF-8" standalone="yes"?> <persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" version="2.1" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd"> <persistence-unit name="my-persistence-unit"> <description>Hibernate Tips</description> <provider>org.hibernate.jpa.HibernatePersistenceProvider</provider> <exclude-unlisted-classes>false</exclude-unlisted-classes> <properties> <property name="hibernate.dialect" value="org.hibernate.dialect.PostgreSQLDialect" /> <property name="hibernate.use_sql_comments" value="true" /> .... </properties> </persistence-unit> </persistence>
The generated comments are often not useful to find a specific query. You should, therefore, use the org.hibernate.comment query hint to provide your own comment.
I use it in the following example to set the SQL comment for my query to “This is my comment”.
TypedQuery q = em.createQuery(“SELECT a FROM Author a WHERE a.id = :id”, Author.class); q.setParameter(“id”, 1L); q.setHint(“org.hibernate.comment”, “This is my comment”); Author a = q.getSingleResult();
Hibernate adds this comment to the generated SQL statement and writes it to the log file.
08:14:57,432 DEBUG [org.hibernate.SQL] – /* This is my comment */ select author0_.id as id1_0_, author0_.firstName as firstNam2_0_, author0_.lastName as lastName3_0_, author0_.version as version4_0_ from Author author0_ where author0_.id=?
Learn More:
I got into a lot more details about Hibernate’s logging capabilities in Hibernate Logging Guide – Use the right config for development and production.
Hibernate and JPA support a lot more hints than I showed you in this post. I summarized the most interesting ones in 11 JPA and Hibernate query hints every developer should know.
Hibernate Tips Book
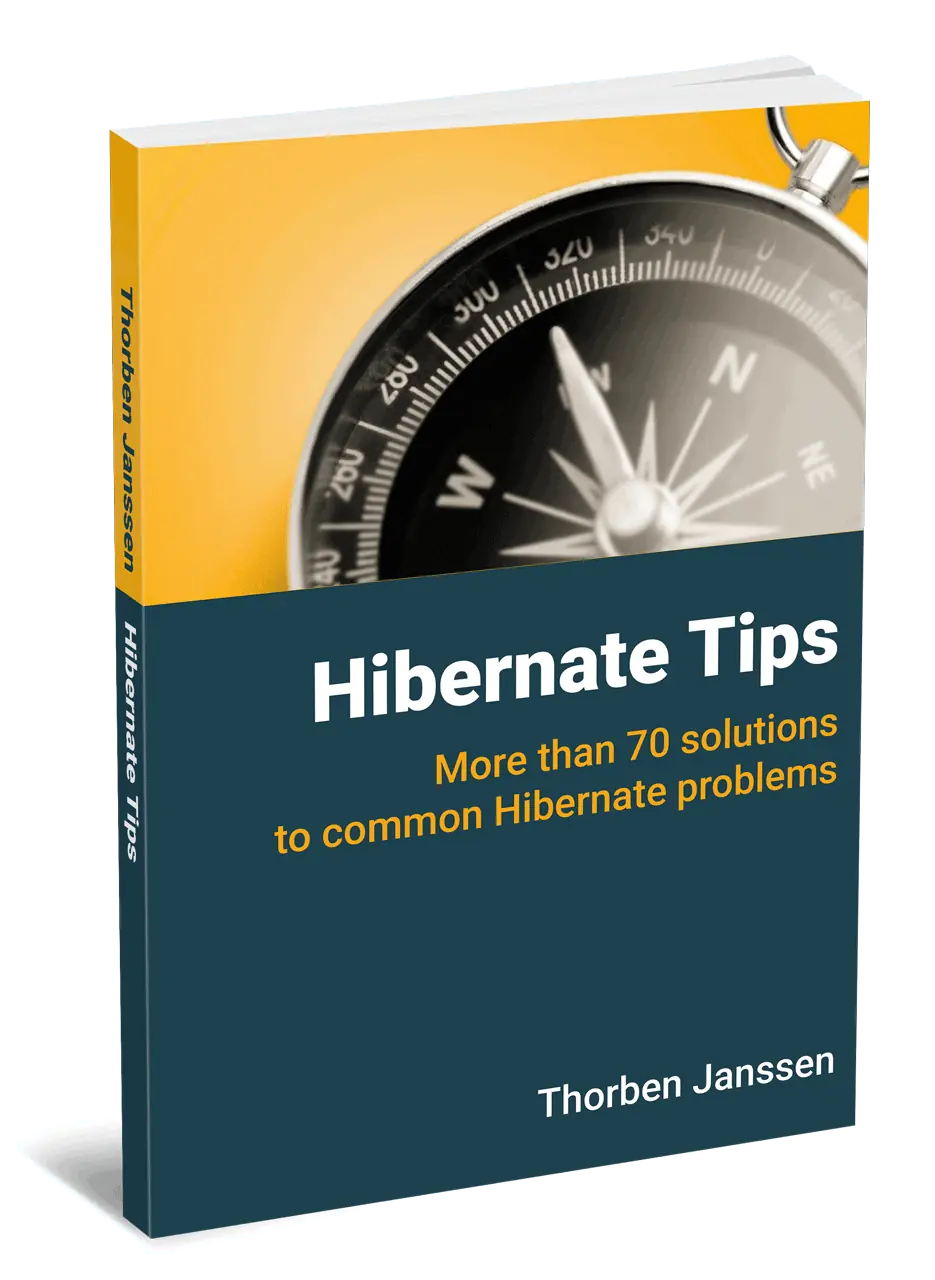
Get more recipes like this one in my new book Hibernate Tips: More than 70 solutions to common Hibernate problems.
It gives you more than 70 ready-to-use recipes for topics like basic and advanced mappings, logging, Java 8 support, caching, and statically and dynamically defined queries.
thank you for giving me wonderful information
Hello,
Thanks for sharing such informative and helpful blog post and you are doing a good job so keep posting such amazing articles
Thanks