Hibernate Tips: How to order the elements of a relationship
Take your skills to the next level!
The Persistence Hub is the place to be for every Java developer. It gives you access to all my premium video courses, monthly Java Persistence News, monthly coding problems, and regular expert sessions.
Hibernate Tips is a series of posts in which I describe a quick and easy solution for common Hibernate questions. If you have a question you like me to answer, please leave a comment below.
Question:
How can I order the elements of an annotate relationship without writing my own query?
Solution:
JPA supports the @OrderBy annotation which you can add to a relationship attribute as you can see in the following code snippet.
@ManyToMany @JoinTable(name="BookAuthor", joinColumns={@JoinColumn(name="bookId", referencedColumnName="id")}, inverseJoinColumns={@JoinColumn(name="authorId", referencedColumnName="id")}) @OrderBy(value = "lastName ASC") private Set<Author> authors = new HashSet<Author>();
In this example, I want to order the Authors who have written a specific book by their last name. You can do this by adding the @OrderBy annotation to the relationship and specifying the ORDER BY statement in its value attribute. In this case, I define an ascending order for the lastName attribute of the Author entity.
If you want to order by multiple attributes, you can provide them as a comma-separated list as you know it from SQL or JPQL queries.
Hibernate uses the value of the annotation to create an ORDER BY statement when it fetches the related entities from the database.
05:22:13,930 DEBUG [org.hibernate.SQL] – select authors0_.bookId as bookId1_2_0_, authors0_.authorId as authorId2_2_0_, author1_.id as id1_0_1_, author1_.firstName as firstNam2_0_1_, author1_.lastName as lastName3_0_1_, author1_.version as version4_0_1_ from BookAuthor authors0_ inner join Author author1_ on authors0_.authorId=author1_.id where authors0_.bookId=? order by author1_.lastName asc
Hibernate Tips Book
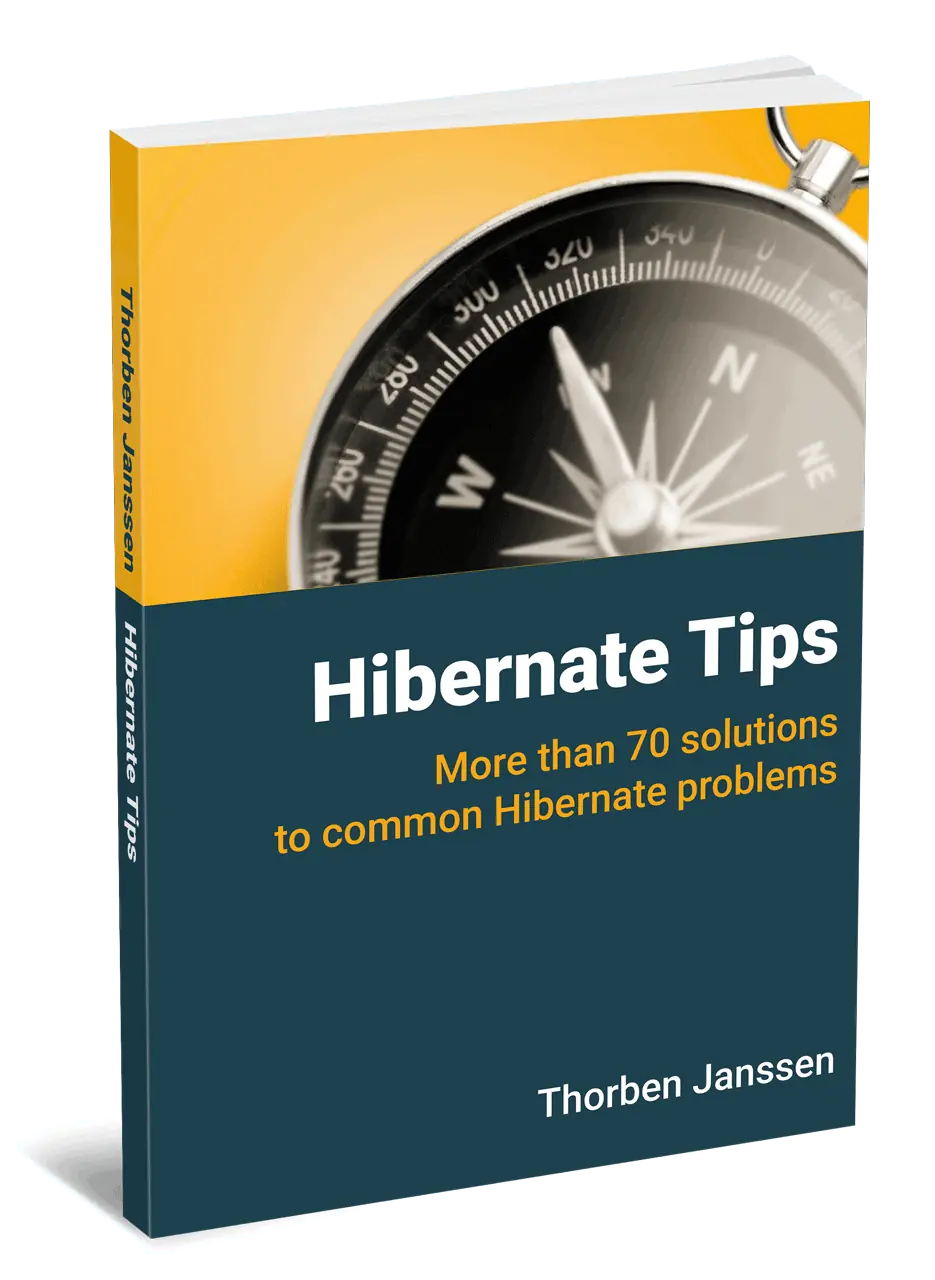
Get more recipes like this one in my new book Hibernate Tips: More than 70 solutions to common Hibernate problems.
It gives you more than 70 ready-to-use recipes for topics like basic and advanced mappings, logging, Java 8 support, caching, and statically and dynamically defined queries.
You are specifying OrderBy over a HashSet. Does it even make any sense?
Hi Keerthi,
Yes, it makes sense because the HashSet gets only used when you instantiate a new entity object. When you read it from the database, Hibernate uses its own Set implementation, which keeps the defined order.
Regards,
Thorben
If the @OrderBy column is _not_ specified, will the association use one of the join columns to order by implicitly, or will the ordering of the result set potentially be non-deterministic?
If you don’t specify the order, then it’s undefined. That means it depends on the internal implementation of your database and might not be deterministic.