Hibernate Tips: How to map a Boolean to Y/N
Take your skills to the next level!
The Persistence Hub is the place to be for every Java developer. It gives you access to all my premium video courses, monthly Java Persistence News, monthly coding problems, and regular expert sessions.
Hibernate Tips is a series of posts in which I describe a quick and easy solution for common Hibernate questions. If you have a question for a future Hibernate Tip, please post a comment below.
Question:
I’m working with a legacy database that stores booleans as the characters ‘Y’ and ‘N’. What’s the easiest way to map these values to an entity attribute of type Boolean?
Solution:
By default, Hibernate maps entity attributes of type Boolean to a database column of type boolean. You can change that by implementing an AttributeConverter that maps the Boolean to a Character. Hibernate will transparently apply this converter whenever it uses the entity attribute in an SQL INSERT, UPDATE or SELECT statement.
The implementation of such a converter is pretty easy. You just need to implement the AttributeConverter<Boolean, Character> interface with its methods convertToDatabaseColumn and convertToEntityAttribute. Within these methods, you implement the conversion of the Boolean object to a Character object and vice versa.
You also need to annotate your class with a @Converter annotation. This registers the AttributeConverter, and you can use the autoApply attribute to define if the converter shall be used for all attributes of the converted type.
OK, let’s take a look at an AttributeConverter that converts a Boolean to a Character.
@Converter(autoApply = true) public class BooleanConverter implements AttributeConverter<Boolean, Character> { @Override public Character convertToDatabaseColumn(Boolean attribute) { if (attribute != null) { if (attribute) { return 'Y'; } else { return 'N'; } } return null; } @Override public Boolean convertToEntityAttribute(Character dbData) { if (dbData != null) { return dbData.equals('Y'); } return null; } }
As you can see, the implementation of such a converter doesn’t require a lot of code. There are nevertheless 2 things you should pay attention to:
- I annotated the BooleanConverter class with the @Converter annotation and set the autoApply attribute to true. This tells Hibernate to apply this converter to all entity attributes of type Boolean.
- Entity attributes and database columns can contain null values, and you need to handle them in your convertToDatabaseColumn and convertToEntityAttribute methods.
That’s all you need to do to persist an entity attribute of type Boolean as the characters ‘Y’ and ‘N’. As soon as you add the AttributeConverter to your application, Hibernate will use it to convert the matching entity attributes and database columns in all SQL statements.
Learn more:
If you want to learn more about AttributeConverter and other options to map custom data types, you might enjoy reading the following articles:
- How to implement a JPA Attribute Converter
- JPA 2.1 Attribute Converter – The better way to persist enums
- How to use PostgreSQL’s JSONB data type with Hibernate
Hibernate Tips Book
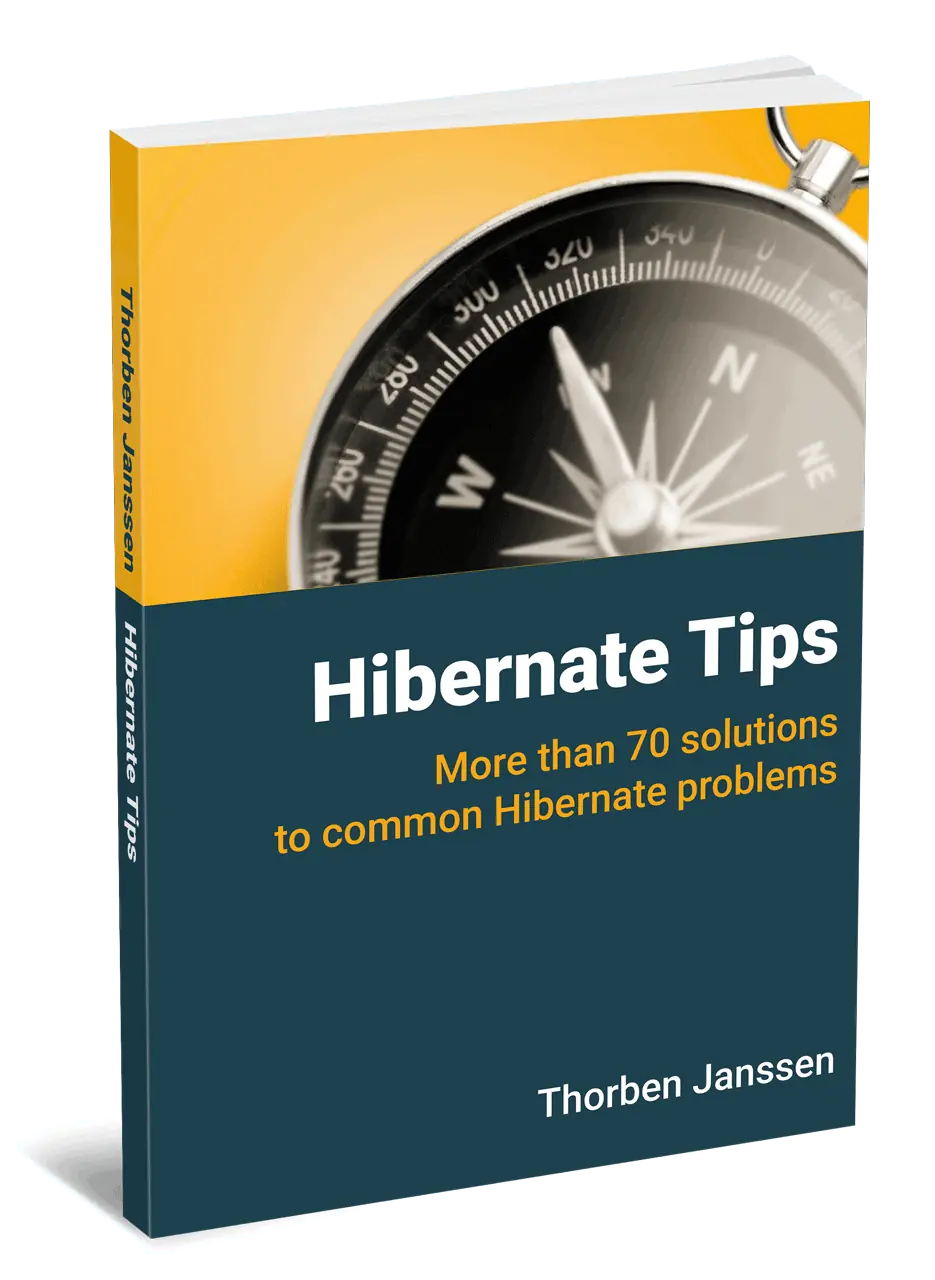
Get more recipes like this one in my new book Hibernate Tips: More than 70 solutions to common Hibernate problems.
It gives you more than 70 ready-to-use recipes for topics like basic and advanced mappings, logging, Java 8 support, caching, and statically and dynamically defined queries.